
Some commands
mkdir AngularLearning
cd AngularLearning/
node --version
v10.3.0
bower --version
1.8.2
npm --version
6.1.0
npm install express
sudo npm install -g npm
bower install angular
bower install bootstrap
sudo mkdir public → cd public → sudo mkdir src → sudo mkdir view
How to run UI of pringleExtra project
Clone from
npm install
bower install
grunt serve
now automatically it will open in browser
Location of UI project in S3: dev2.apps.thermofisher.com/apps/pringles-xtra
Structure of Angular Project.
e2e: end to end testing using
node module : dependencies(will not upload to server)
Assets : images/GIF
Environment : PROD/TEST etc
main.ts (type script) : starting point of the application (like main in java)
polifils : fill the gap of javascript and browser
test.ts : setting test env
editorconfig: for team everyone has to be same file
package.json : every nodejs project have (library of angular)
tsconfig.json : typescript compile.
tslint.json static analysis code readability maintainability and functionality of the typescript code
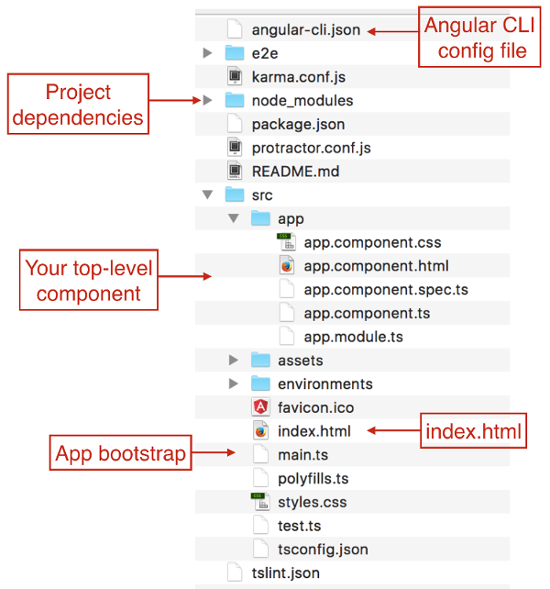
Before Angular we shd know about TS typescript


For installing type script : sudo npm install -g typescript
tsc --version
Launching from the command line
You can also run VS Code from the terminal by typing 'code' after adding it to the path:
- Launch VS Code.
- Open the Command Palette (⇧⌘P) and type 'shell command' to find the Shell Command: Install 'code' command in PATH command.

- Restart the terminal for the new
$PATH
value to take effect. You'll be able to type 'code .' in any folder to start editing files in that folder.
main.ts
function log(message)
{
console.log(message);
}
var message = "Hello Narendra";
{
console.log(message);
}
var message = "Hello Narendra";
log(message);
compile your code using tsc main.ts after compilation main.js will come to the folder (like .java to .class)


Difference between let and var in typescript (var is globle scope in the function but let is limited to the function and let is better for good programming practice)
After compilation let will became var only and will not through any compilation error while compiling with let (like below pic)


Valid in javaScript but not in TypeScript
let count = 5;
count ='1';
Types in TS

CLASS in TS
Run using
tsc main.ts && node main.js
class Test { x: number; y: string; draw() { console.log('Narendra class' + this.x + this.y); } } let test = new Test(); test.x = 1; test.y = 'Raguhwanshi'; test.draw(); |
Constructor ,Class, Interface, Properties, Object , Access modified will be same as Java programming with more flexibility
Here is example of Module

Now Get started with AngularJS
Component basic building block of the angularApplication where we place the data html template and logic
and Module is collection of component in big application (for small app we will have only app module)
Steps to create a component :
- create a component
- register it in module
- Add element in HTML markup
Know the difference between Observables vs Promises in latest angular
get the dummy API from https://jsonplaceholder.typicode.com/posts
get the data from above API and play with it.
Take care of below component

Angular is MVW :

Shotcuts
> ng generate component nav
// output
> ng g c about
// output
> ng g c contact
// output
> ng g c home
// output